拖动
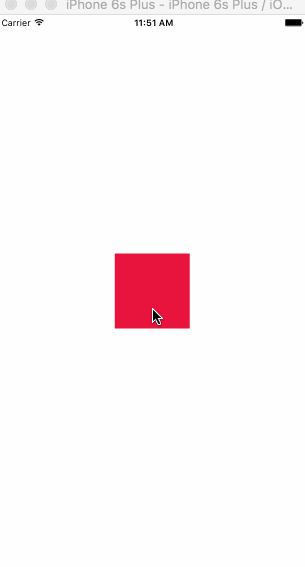
import React from 'react';
import {
View,
StyleSheet,
PanResponder,
Dimensions,
Animated
} from 'react-native';
const {
height: deviceHeight,
width: deviceWidth
} = Dimensions.get('window');
export default class DragSquare extends React.Component {
constructor(props) {
super(props);
this.state = {
animatedValue: new Animated.Value(0)
}
}
componentWillMount() {
this._animatedValue = new Animated.ValueXY();
this._value = {x: 0, y: 0};
this._animatedValue.addListener((value) => this._value = value);
this._panResponder = PanResponder.create({
onStartShouldSetPanResponder: (evt, gestureState) => true,
onStartShouldSetPanResponderCapture: (evt, gestureState) => true,
onMoveShouldSetPanResponder: (evt, gestureState) => true,
onMoveShouldSetPanResponderCapture: (evt, gestureState) => true,
onPanResponderGrant: (e, gestureState) => {
this._animatedValue.setOffset({x: this._value.x, y: this._value.y});
},
onPanResponderMove: Animated.event([
null, {dx: this._animatedValue.x, dy: this._animatedValue.y}
]),
onPanResponderRelease: () => {
this._animatedValue.flattenOffset();
}
});
}
componentDidMount() {
}
render() {
let interpolatedColor = this._animatedValue.y.interpolate({
inputRange: [0, deviceHeight - 100],
outputRange: ['rgba(229, 27, 66, 1)', 'rgba(90, 146, 253, 1)'],
extrapolate: 'clamp'
});
let interpolatedRotation = this._animatedValue.x.interpolate({
inputRange: [0, deviceWidth/2, deviceHeight],
outputRange: ['-360deg', '0deg', '360deg']
});
return (
<View style={styles.container}>
<Animated.View style={[
styles.box,
{
transform: [
{translateX: this._animatedValue.x},
{translateY: this._animatedValue.y},
{rotate: interpolatedRotation}
],
backgroundColor: interpolatedColor
}
]} {...this._panResponder.panHandlers}/>
</View>
);
}
}
var styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center'
},
box: {
width: 100,
height: 100
}
});